Here is my sample UDP socket code written in C. Unlike other versions you get commonly on-line this version is focused towards systems and network software developers and as a quick reference even for experienced software programmers. In this example/template, I am sending test raw binary data bytes from UDP sample client to registered UDP sample server. The code has documentation already in the form of comments (as you can see below). You can download my source below and you can use it directly in your projects or you can use the same for learning purposes to understand about user-space socket programming via IPv4 UDP transport layer protocol.
UDP is very unique, versatile and flexible unlike TCP, hence this can be used as internal device IPC mechanism instead using more traditional platforms such as message queues, pipes, etc. Unlike other IPC mechanisms, this provides a transparent fool-proof way to debug your software via Wireshark packet capture and allows you to fix the issues by going through the raw binary packet capture data.
UDP as a future high-speed network communication platform: UDP is also experimented to be used as a high-speed communication career protocol unlike TCP. TCP restricts advance Network software architects to use TCP provided congestion control, out-of-order packet sequence, features. But UDP provides a raw access to the network, so that advance users can architect these utilities in the user-space, application layer protocol(s). For example for the same obvious reasons Google invented QUIC protocol, which uses UDP as its career protocol in Google’s Chrome browser instead TCP based HTTPS/SSL protocol. QUIC has its own user-space application layer reliability layer which ensures situations like packet loss, security, congestion control and so on. And with QUIC Google can also multiplex multiple remote connections/sessions into one communication channel there by improving network performance and user experience.
Here is my very detailed live demo video of the same, if you are interested kindly watch my video so that you can get the full bigger perspective. I have also discussed all the above points in-depth.
Here is my source-code of my test UDP socket client.
/* udp_socket_client.c
* The Linux Channel
* Author: Kiran Kankipati
* Updated: 01-Feb-2017
*/
#include <stdio.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#define MAXBUF 1024
int main()
{ int sockfd, len, i;
unsigned char buf[MAXBUF];
struct sockaddr_in udp_server_addr;
socklen_t addr_size;
sockfd = socket(PF_INET, SOCK_DGRAM, 0); // create a UDP socket
if(sockfd<=0) { printf("socket error !\n"); return 0; }
/* configure settings to communicate with remote UDP server */
udp_server_addr.sin_family = AF_INET;
udp_server_addr.sin_port = htons(6800); // server port
udp_server_addr.sin_addr.s_addr = inet_addr("127.0.0.1"); // local-host
memset(udp_server_addr.sin_zero, '\0', sizeof udp_server_addr.sin_zero);
addr_size = sizeof udp_server_addr;
memset(buf, 0xab, MAXBUF); // set the entire buffer with 0xab (i.e 1010 1011 binary)
sendto(sockfd, buf, MAXBUF, 0, (struct sockaddr *)&udp_server_addr, addr_size); //send the data to server
len = recvfrom(sockfd, buf, MAXBUF, 0, NULL, NULL); // receive data from server
printf("Received from server: %d bytes\n", len); for(i=0;i<len;i++) { printf("%02x ", buf[i]); } printf("\n"); //print received data dump
close(sockfd); //close socket file-descriptor
return 0;
}
Compile the udp_socket_client.c:
kiran@WD-1TB2:/code/thelinuxchannel/udp_socket$ gcc -o udp_socket_client udp_socket_client.c
kiran@WD-1TB2:/code/thelinuxchannel/udp_socket$
Here is my source-code of my test UDP socket server.
/* udp_socket_server.c
* The Linux Channel
* Author: Kiran Kankipati
* Updated: 01-Feb-2017
*/
#include <stdio.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#define MAXBUF 1024
int main()
{ int sockfd, len, i;
unsigned char buf[MAXBUF];
struct sockaddr_in udp_server_addr;
struct sockaddr_in udp_client_addr;
struct sockaddr_storage server_storage;
socklen_t addr_size, client_addr_size;
sockfd = socket(PF_INET, SOCK_DGRAM, 0); // create a UDP socket
if(sockfd<=0) { printf("socket error !\n"); return 0; }
/* configure settings to register UDP server */
udp_server_addr.sin_family = AF_INET;
udp_server_addr.sin_port = htons(6800); // server port
udp_server_addr.sin_addr.s_addr = inet_addr("127.0.0.1"); // local-host
memset(udp_server_addr.sin_zero, '\0', sizeof udp_server_addr.sin_zero);
bind(sockfd, (struct sockaddr *) &udp_server_addr, sizeof(udp_server_addr)); // bind the socket
addr_size = sizeof server_storage;
len = recvfrom(sockfd, buf, MAXBUF, 0, (struct sockaddr *)&server_storage, &addr_size); //receive data from client
printf("Received from server: %d bytes\n", len); for(i=0;i<len;i++) { printf("%02x ", buf[i]); } printf("\n"); //print received data dump
memset(buf, 0xcd, MAXBUF); // set the entire buffer with 0xcd (i.e 1100 1101 binary)
sendto(sockfd, buf, MAXBUF, 0, (struct sockaddr *)&server_storage, addr_size); //send the data to client
return 0;
}
Compile the udp_socket_server.c:
kiran@WD-1TB2:/code/thelinuxchannel/udp_socket$ gcc -o udp_socket_server udp_socket_server.c
kiran@WD-1TB2:/code/thelinuxchannel/udp_socket$
Execute the binaries:
kiran@WD-1TB2:/code/thelinuxchannel/udp_socket$ ls
udp_socket_client udp_socket_client.c udp_socket_server udp_socket_server.c
kiran@WD-1TB2:/code/thelinuxchannel/udp_socket$
First execute the udp_socket_server in one terminal so that it registers and listens on the port 6800 as shown below:
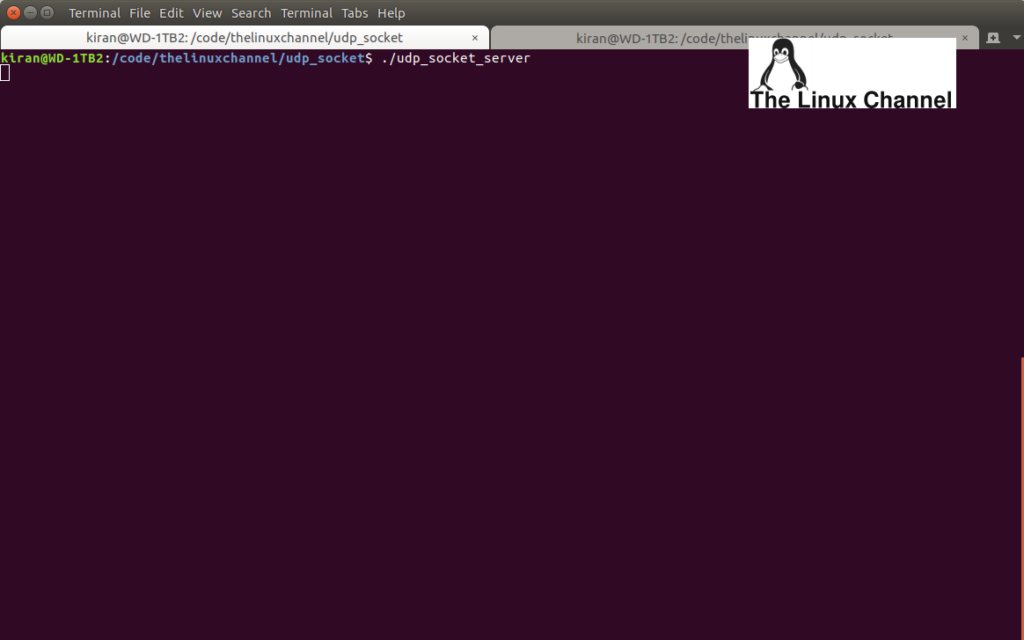
Now on a different terminal check the status of the UDP server registration via netstat -l command:
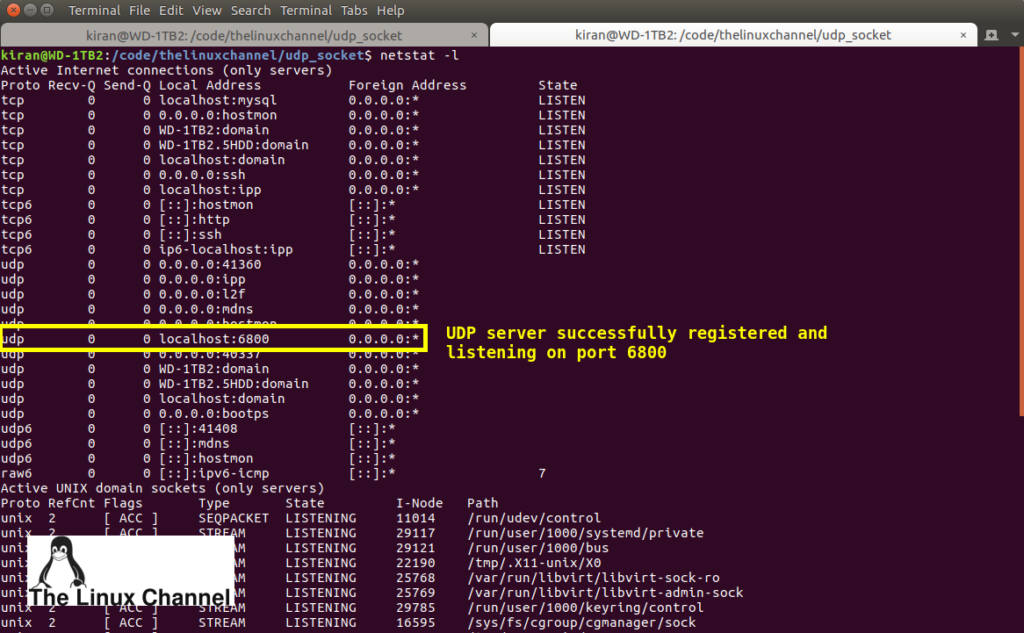
Before executing the UDP client, open Wireshark and capture the local loopback port lo as shown below:
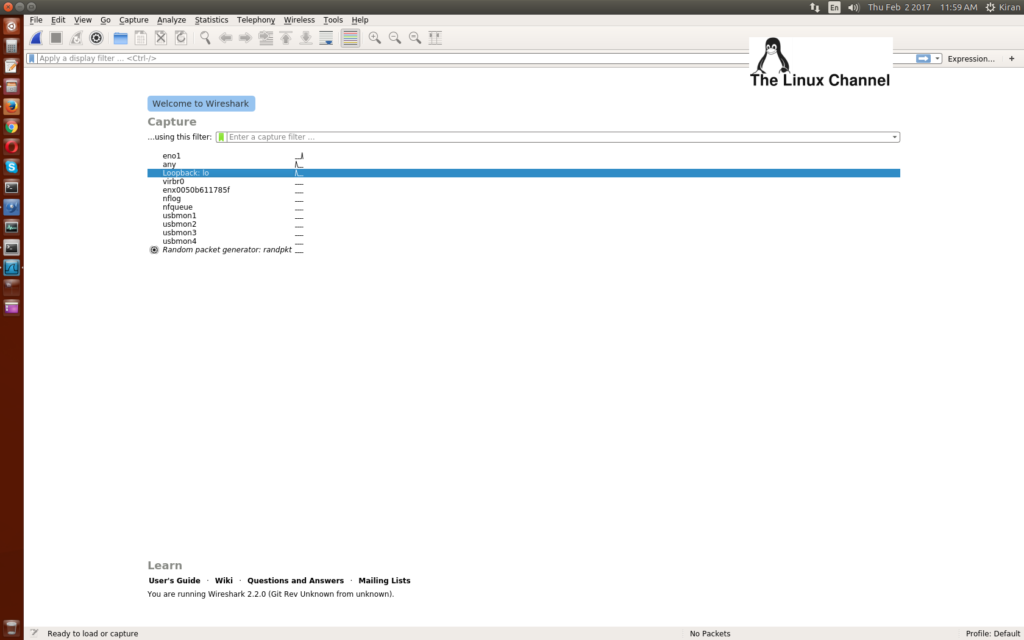
Execute the UDP socket client on a different terminal as shown below. You can see as soon as it gets executed, it sends the test data to the UDP socket server and receives the test data from the server.
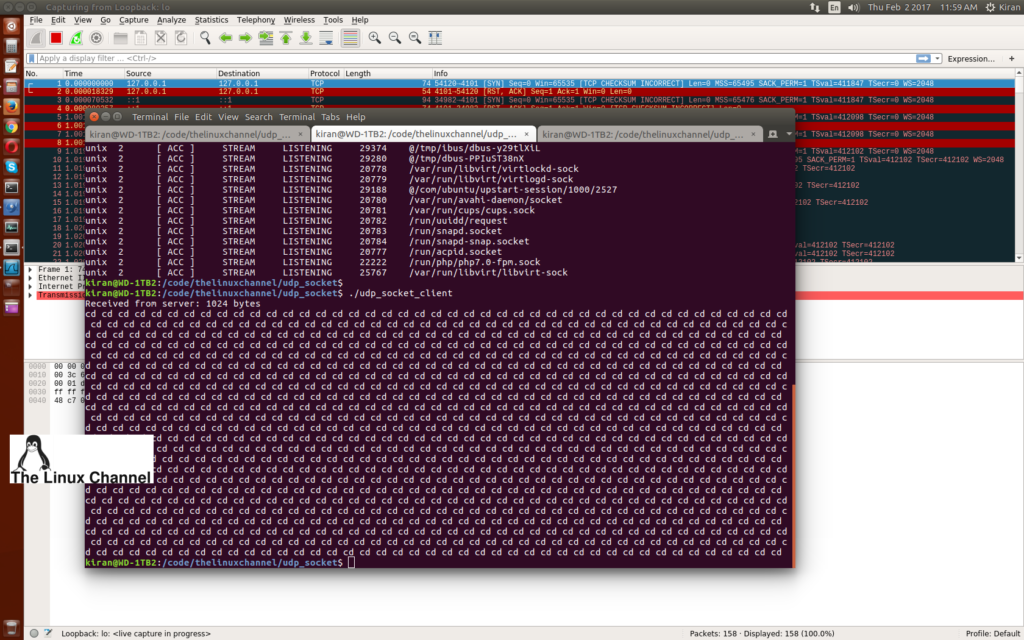
Here is the screenshot of the UDP socket server execution terminal. UDP socket server receives the test data from client prints the same on the screen (as shown below) and quickly responds to the client by sending its own test data dump.
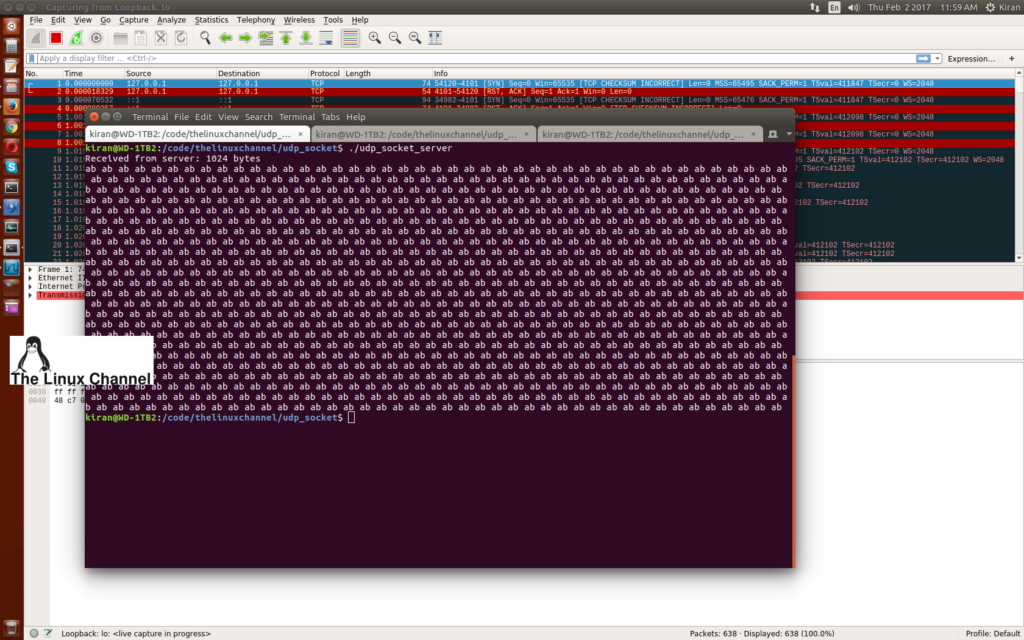
In the Wireshark packet capture you can see these packets, analyse and confirm the same. Below is the Wireshark packet capture of UDP packet from client to server:
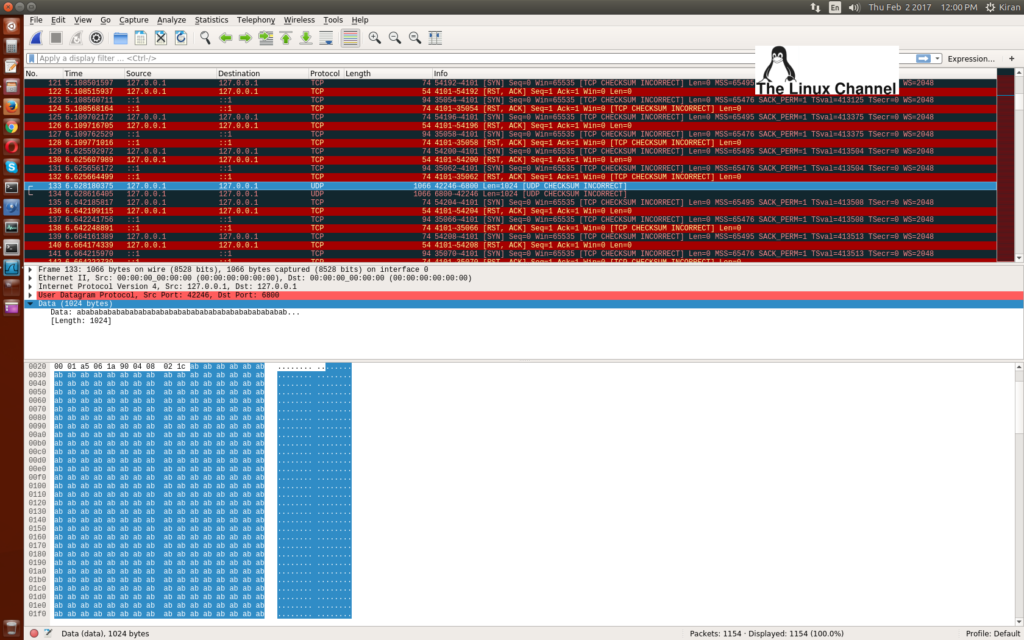
And here is the Wireshark packet capture of UDP packet from server to client:
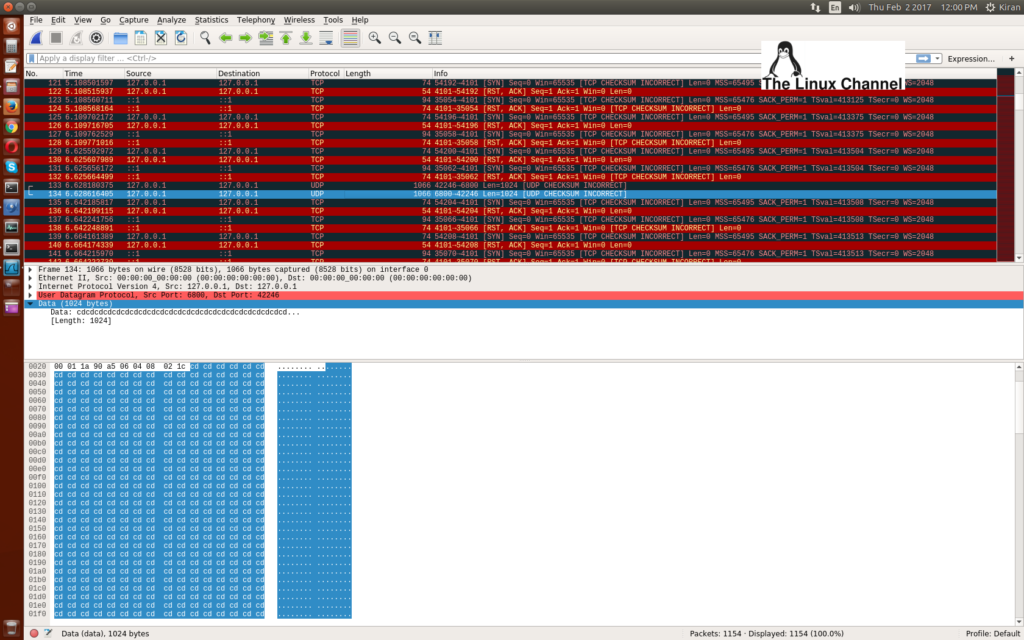
Linux Courses Offered: If you are interested to join classes on Networking, Linux, Systems software, etc with live demo training sessions. You can learn via distance learning platform. I teach my students in old fashioned way the “Guru-Shishya” model. And I believe this is how the education should be provided with no strings attached and boundaries. For more details click HERE.
Download my UDP socket complete sample code (discussed above) which I hosted in my GitHub Repo.
If you have any queries or anything to discuss further on Linux Kernel Programming and writing Kernel modules kindly feel free to contact me.